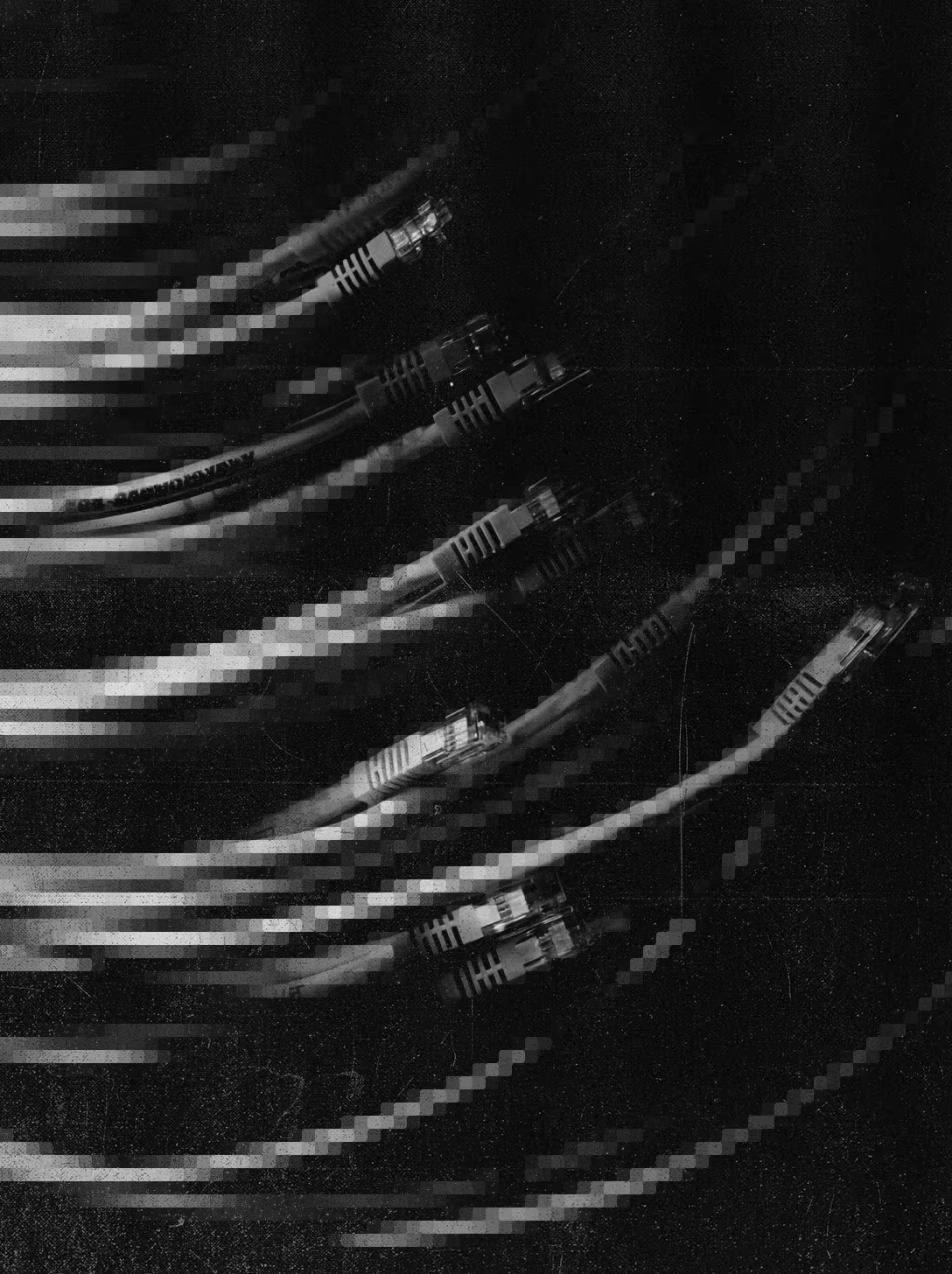
How SkipLabs is building React for the backend
How SkipLabs is building React for the backend
In 2013, Facebook open-sourced React, a JavaScript library for building user interfaces. React’s foundational idea was that developers describe what the UI should look like, and React handles the how efficiently updating the DOM (the data representation of the objects that comprise the structure and content of a web page) leveraging an innovation called the Virtual DOM. When the state of any front-end component changes, React calculates the difference between the previous and current Virtual DOM and updates only the necessary parts of the UI, always keeping it in sync with the underlying data.
React was a radical departure from the status quo of imperative UI frameworks. The state of the art at the time was jQuery, which required developers to explicitly instruct the browser how to update the UI in response to events or data changes. React was a completely different way of thinking about things, and required real evangelism to convert skeptics. But once devs made the leap, it became clear that the declarative, component-based philosophy greatly simplified frontend development and improved performance. Today React is the most popular web framework among professional developers.
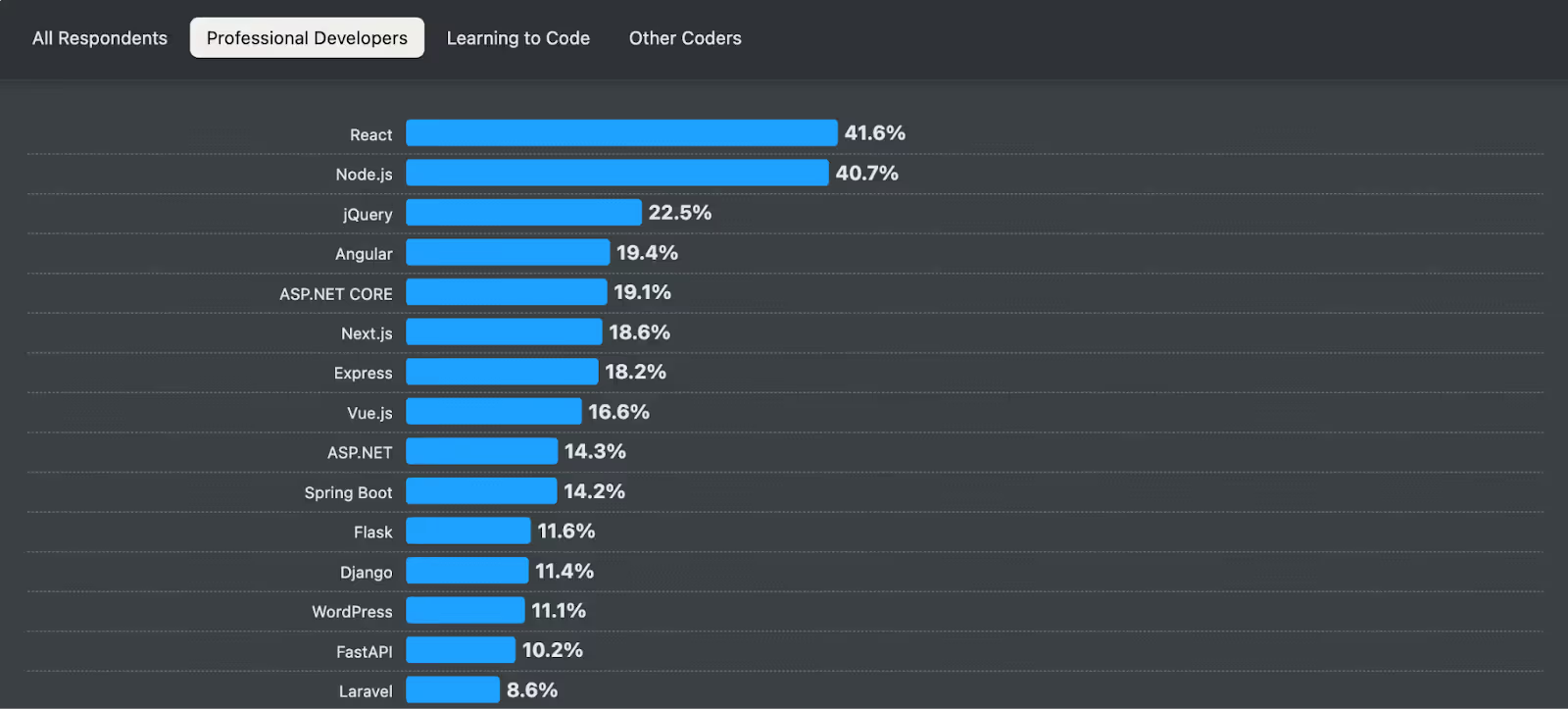
But what about the backend? In a microservices-oriented world, a single application may be composed of dozens—or even hundreds—of discrete services, orchestrating interactions with tens of thousands of clients and third-party APIs, all continuously reading and writing data. State management, once a more tractable concern, has exploded into an intricate web of dependencies, introducing an overwhelming degree of complexity.
Solving state today is akin to navigating the treacherous waters of the Cape of Good Hope—many talented developers have been lost to its challenges. The fundamental difficulty lies in the sheer unpredictability and fragmentation of modern distributed systems. Services must coordinate over unreliable networks, handle concurrent updates, and gracefully recover from failures—all while ensuring consistency, availability, and performance. Developers wrestle with race conditions, stale reads, eventual consistency trade-offs, and the ever-present risk of data corruption when systems fall out of sync.
Traditional databases and caches offer partial solutions, but they often require brittle, custom logic to reconcile different sources of truth (you know what they say about cache invalidation). Meanwhile, event-driven architectures introduce another set of challenges: handling duplicate messages, ensuring idempotency, and replaying state correctly when things go wrong. As systems scale, the combinatorial complexity of state management grows exponentially, making it difficult for teams to maintain reliability without sacrificing agility. Essentially, there’s no silver bullet: building a scalable backend is hard work.
To extend the earlier metaphor, what developers need is a sturdier vessel, purpose-built to weather these treacherous waters. That’s where SkipLabs comes in.
SkipLang and reactive programming
Before diving into the nuts and bolts of how Skip promises to revolutionize backend development—much like React transformed the frontend—let’s take a step back in time and go, once again, to Facebook in the 2010s.
While technologies like React, GraphQL and PyTorch get a lot more buzz, few of Facebook’s innovations drove more internal developer productivity than Hack. Hack was a dialect of PHP that introduced the idea of gradual typing, allowing developers to code quickly, while also having safety features built in, like static typechecking. Much of the Facebook app is still written in Hack.
The brain behind Hack was Julien Verlaguet, described to us as one of the top two or three programming language designers in the world. One day, as Julien was designing Hack’s typechecker, he realized he needed snappier performance; a tool that would react to changes in real-time. Simultaneously, Facebook was in the process of re-designing their privacy layer that required navigating a menagerie of complex permissions logic.
And that’s when the idea for a new programming language called Skip coalesced.
The catchy description of Skip is that it’s a language that “skips” over the things you have already computed. What that practically means is Skip introduces an intelligent way to handle caching automatically updating stored results when data changes. It does this carefully tracking which parts of a program can change and which are stable. If Skip can guarantee that a function doesn’t cause unexpected changes, developers can safely store (or "memoize") its results, and Skip will automatically refresh them when the underlying data updates. This makes applications a lot faster and more efficient, and frees developers from having to manually manage cache updates.
We met Julien shortly after Skip was open sourced, and could tell that he viscerally felt that the future of backend development was Skip. Plenty of heavy hitters opined the same.

But Skiplang didn’t quite catch fire inside Facebook like React and Hack had. So Julien left the company and embarked on a several year excursion to figure out he could make declarative backend engineering more approachable and consumable for developers outside of Facebook. Just a few weeks ago, his team launched their v1 of the Skip Framework.
The Skip Framework: state management on autopilot
Written in SkipLang, Skip is an open-source framework designed to make it incredibly easy to build reactive backend systems. It does for backends what React did for frontends: providing a declarative, simple-yet-comprehensive abstraction with a Typescript interface for building real-time features. Skip allows developers to write application logic that automatically updates in response to changes, eliminating the need for manual dependency tracking, cache invalidation logic, or hand-coding complex state management.
For a quick example, in my capacity as Assistant to The Traveling Secretary, everyone in my friend group wants to know who is in Jerry’s apartment at any point in time (so we can all hang out). But we also want to make sure that we only see friends there, and not our enemies (like Newman). We can create a few primitives in Skip to represent the apartment and our friends:
type PersonID = ...;
type Person = { name: string, friends: PersonID[], enemies: PersonID[]... }
type Apartment = { members: PersonID[], ... }
Note that each person has a list of friends and enemies that are in constant flux.
The complexity here is: how do we make sure that the apartment object is dynamically updated when who is in it (friends coming in and out), as well as who we want to see there (who is on our friends and enemies lists), is constantly changing? Skip’s reactive computation graph deals with all of this complexity for you. All you need to do is write the logic for who is in which groups, and then let Skip do the rest:
const instance = await initService({
initialData: { persons, apartment },
resources: { friends: Friends },
createGraph(input: ServiceInputs): ResourceInputs {
const actives = input.groups.map(FriendsInApartment, input.persons);
return { users: input.persons, actives };
}});
const service = runService(instance);
With Skip, applications remain responsive and always up-to-date allowing engineers to focus on building features rather than managing state changes.
A key lesson from React’s success is that developers should be able to declaratively specify the desired state of their application, while the underlying runtime handles the coordination and synchronization required to ensure responsiveness and consistency.
Much in that same vein, developers write Skip services in a declarative style, defining a reactive computation graph over some input data. Under the hood, the Skip framework efficiently evaluates and updates computations, delivering always up-to-date and correct results without incurring heavy recomputation costs or requiring any bug-prone explicit handling of dependency tracking or change propagation.
Skip ultimately enables developers to put their state management layer on autopilot and build snappy, real-time features. Like React, it requires a shift in thinking, but it’s similarly game-changing.
With that, we’re thrilled to announce that Amplify has led Skip Labs’s Series Seed. We’re beyond excited to support Julien and his amazing team’s mission to do for backend development what React has done to the frontend.
Skip is completely open source. You can install it via npm:
npm install @skipruntime/core @skipruntime/server @skipruntime/helpers
Check out their docs here, take a look at some reactive examples, and learn more about their story on the site.